How to setup Tryon in model mode?
Model Mode Tryon allows users to virtually try on products in real-time using augmented reality. This feature can significantly enhance the user experience by providing a realistic preview of products such as jewelleries, watches and more. Follow these steps to set up Model Mode Tryon using our Web SDK.
Prerequisites
Before you begin, ensure you have the following:
- Basic Knowledge of JavaScript/TypeScript: Familiarity with JavaScript or TypeScript is essential.
- Node.js and npm/yarn Installed: You will need Node.js and npm or yarn installed on your machine to manage dependencies.
- Unique Brand Key: Obtain a brand ID key from your mirrAR POC.
- A Model Image: You will use this image for face or hand tracking.
Basic flow
Here is the basic flow of operations that will be done to use the live tryon.
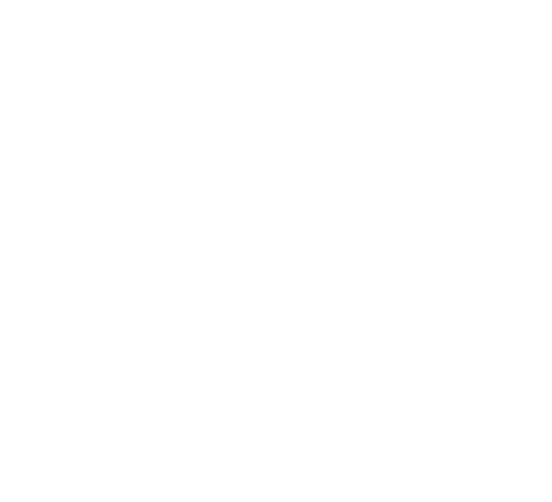
Integration Steps
You can follow the below steps to integrate the model mode directly into your web application.
Step 1: Installation
To begin, you need to install the AR Engine and Gallery/Model Mode module from the collection of our modules. You can install them using npm.
npm install @mirrar-sdm/ar-engine @mirrar-sdm/gallery-mode
Step 2: Setup the Model/Gallery mode
<div id="ar-container">
<canvas id="modelCanvas" width="640" height="480"></canvas>
</div>
import { GalleryMode } from '@mirrar-sdm/gallery-mode'
const imageURL = 'model image url'
const canvas = document.getElementById('modelCanvas')
GalleryMode.setModelURL(imageURL)
GalleryMode.init(canvas)
setupAREngine(canvas)
Step 3: Setup & Initialize the AR Engine
import {AR_Engine} from "@mirrar-sdm/ar-engine"
import { TrackingTypeEnum } from "@mirrar-sdm/ar-engine/lib/TrackingManagers/MachineLearningTrackingManager"
function setupAREngine(canvas) {
const container = document.getElementById('ar-container')
// You can set any dimensions here but it is advised
// to set the dimensions of AR Engine same as camera feed
const options = {
container: container,
dimensions: {
width: canvas.width,
height: canvas.height
}
}
const arEngine = new AR_Engine(options)
}
Step 4: Setup Face or Hand Tracking depending on the type of jewellery that you want to augment.
// choose tracking type as face or hand
const trackingType = TrackingTypeEnum.Face // TrackingTypeEnum.Hand
// function to get the download progress of the AI model
const progressFunction = (values) -> {
console.log("Download Progress", values)
}
// callback that will be called after completion of Face/Hand Tracking Setup
const onComplete = () => {
console.log("Tracking setup successfull")
}
arEngine.setupTracking(trackingType, progressFunction, (data) => {
onComplete(data)
})
Step 5: Process gallery/model frame in AR Engine
if(arEngine) {
arEngine.processFrame(canvas, onProcessingComplete)
}